음악, 삶, 개발
Juce 로 만드는 GUI 파트8 - Rectangle 클래스 (2개의 Point) 본문
< Rectangle 클래스 >
Rectangle 클래스는 사각형을 x, y, width, height 으로 나타내는 클래스이다.
Rectangle 클래스는 Point 나 Line 클래스처럼 Rectangle<ValueType> 으로 정의되는 template 클래스이다.
Rectangle 클래스 역시 Line 클래스처럼 두개의 Point 객체로 이루어져있지만,
각 Point 객체가 나타내는 지점이 다르다.
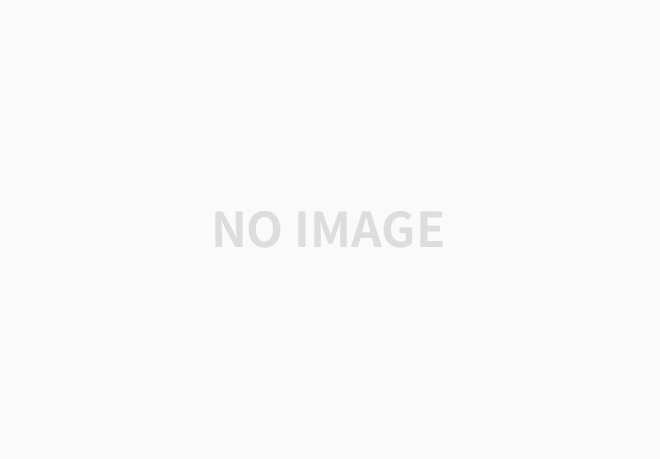
위의 그림처럼, 각 Point 는 왼쪽 상단 모서리와, 오른쪽 하단 모서리의 x, y 좌표를 나타낸다.
두개의 Point 객체를 인자로 넘겨 Rectangle 객체를 생성할수도있고,
또는 x, y, width, height 를 인자로 넘겨서 생성할수도있다.
미리 말하면, Rectangle 클래스는 매우매우 중요하다.
추후, Component 의 paint 함수에서 사각형을 그리는데에 사용되는것뿐만아니라,
Component 의 크기를 결정할때도 인자로 사용된다. (이 사실이 매우 중요)
Rectangle 객체를 인자로 받는 다른 클래스들의 함수들을 추후 배울것이다.
여러 곳에서 다양한 용도로 사용되는것이 Rectangle 클래스이기때문에,
그만큼 100가지에 달하는 멤버 함수를 가지고있다.
따라서, 우리가 Rectangle 객체를 변형하고자할때는,
스스로 자기만의 logic 을 굳이 다시 만들려고하지말고 (Don't reinvent the wheel ! )
반드시 공식문서에 들어가, 내가 찾는 기능을 가진 멤버 함수가 있는지 확인해보기를 바란다.
(사실 우리가 원하는 대부분의 것들이 모두 가능할것이다)
< 공식 문서 >
Rectangle< ValueType > Class Template Reference
Manages a rectangle and allows geometric operations to be performed on it.
See also RectangleList, Path, Line, Point
In function argument :
/* Constructor */
Rectangle () =default
Rectangle (const Rectangle &) =default
Rectangle (ValueType initialX, ValueType initialY, ValueType width, ValueType height) noexcept
Rectangle (ValueType width, ValueType height) noexcept
Rectangle (Point< ValueType > corner1, Point< ValueType > corner2)
/* Destructor */
~Rectangle ()=default
/* Operator */
Rectangle& operator= (const Rectangle& ) =default
Rectangle& operator+= (Point< ValueType > deltaPosition) noexcept
Rectangle& operator-= (Point< ValueType > deltaPosition) noexcept
Rectangle operator*= (FloatType scaleFactor) noexcept
Rectangle operator*= (Point< FloatType > scaleFactor) noexcept
Rectangle operator/= (FloatType scaleFactor) noexcept
Rectangle operator/= (Point< FloatType > scaleFactor) noexcept
Rectangle operator+ (Point< ValueType > deltaPosition) const noexcept
Rectangle operator- (Point< ValueType > deltaPosition) const noexcept
Rectangle operator* (FloatType scaleFactor) const noexcept
Rectangle operator/ (FloatType scaleFactor) const noexcept
bool operator== (const Rectangle& other) const noexcept
bool operator!= (const Rectangle& other) const noexcept
/* Get */
ValueType getX () const noexcept
ValueType getY () const noexcept
ValueType getWidth () const noexcept
ValueType getHeight () const noexcept
ValueType getRight () const noexcept
ValueType getBottom () const noexcept
ValueType getCentreX () const noexcept
ValueType getCentreY () const noexcept
ValueType getAspectRatio (bool widthOverHeight=true) const noexcept
ValueType proportionOfWidth (FloatType proportion) const noexcept
ValueType proportionOfHeight (FloatType proportion) const noexcept
Point< ValueType > getCentre () const noexcept
Point< ValueType > getPosition () const noexcept
Point< ValueType > getTopLeft () const noexcept
Point< ValueType > getTopRight () const noexcept
Point< ValueType > getBottomLeft () const noexcept
Point< ValueType > getBottomRight () const noexcept
Point< ValueType > getConstrainedPoint (Point< ValueType > point) const noexcept
Point< ValueType > getRelativePoint (FloatType relativeX, FloatType relativeY) const noexcept
Range< ValueType > getHorizontalRange () const noexcept
Range< ValueType > getVerticalRange () const noexcept
Rectangle getProportion (Rectangle< FloatType > proportionalRect) const noexcept
Rectangle getIntersection (Rectangle other) const noexcept
Rectangle getUnion (Rectangle other) const noexcept
Rectangle< int > getSmallestIntegerContainer ()
/* Set */
void setPosition (Point< ValueType > newPos) noexcept
void setPosition (ValueType newX, ValueType newY) noexcept
void setSize (ValueType newWidth, ValueType newHeight) noexcept
void setBounds (ValueType newX, ValueType newY, ValueType newWidth, ValueType newHeight) noexcept
void setX (ValueType newX) noexcept
void setY (ValueType newY) noexcept
void setWidth (ValueType newWidth) noexcept
void setHeight (ValueType newHeight) noexcept
void setCentre (ValueType newCentreX, ValueType newCentreY) noexcept
void setCentre (Point< ValueType > newCentre) noexcept
void setHorizontalRange (Range< ValueType > range) noexcept
void setVerticalRange (Range< ValueType > range) noexcept
void setLeft (ValueType newLeft) noexcept
void setTop (ValueType newTop) noexcept
void setRight (ValueType newRight) noexcept
void setBottom (ValueType newBottom) noexcept
void translate (ValueType deltaX, ValueType deltaY) noexcept
void expand (ValueType deltaX, ValueType deltaY) noexcept
void reduce (ValueType deltaX, ValueType deltaY) noexcept
Rectangle removeFromTop (ValueType amountToRemove) noexcept
Rectangle removeFromLeft (ValueType amountToRemove) noexcept
Rectangle removeFromRight (ValueType amountToRemove) noexcept
Rectangle removeFromBottom (ValueType amountToRemove) noexcept
bool enlargeIfAdjacent (Rectangle other) noexcept
bool reduceIfPartlyContainedIn (Rectangle other)
/* Create */
Rectangle withX (ValueType newX) const noexcept
Rectangle withY (ValueType newY) const noexcept
Rectangle withRightX (ValueType newRightX) const noexcept
Rectangle withBottomY (ValueType newBottomY) const noexcept
Rectangle withPosition (ValueType newX, ValueType newY) const noexcept
Rectangle withPosition (Point< ValueType > newPos) const noexcept
Rectangle withZeroOrigin () const noexcept
Rectangle withCentre (Point< ValueType > newCentre) const noexcept
Rectangle withWidth (ValueType newWidth) const noexcept
Rectangle withHeight (ValueType newHeight) const noexcept
Rectangle withSize (ValueType newWidth, ValueType newHeight) const noexcept
Rectangle withSizeKeepingCentre (ValueType newWidth, ValueType newHeight) const noexcept
Rectangle withLeft (ValueType newLeft) const noexcept
Rectangle withTop (ValueType newTop) const noexcept
Rectangle withRight (ValueType newRight) const noexcept
Rectangle withBottom (ValueType newBottom) const noexcept
Rectangle withTrimmedLeft (ValueType amountToRemove) const noexcept
Rectangle withTrimmedRight (ValueType amountToRemove) const noexcept
Rectangle withTrimmedTop (ValueType amountToRemove) const noexcept
Rectangle withTrimmedBottom (ValueType amountToRemove) const noexcept
Rectangle translated (ValueType deltaX, ValueType deltaY) const noexcept
Rectangle expanded (ValueType delta) const noexcept
Rectangle expanded (ValueType deltaX, ValueType deltaY) const noexcept
Rectangle reduced (ValueType delta) const noexcept
Rectangle reduced (ValueType deltaX, ValueType deltaY) const noexcept
Rectangle constrainedWithin (Rectangle areaToFitWithin) const noexcept
Rectangle transformedBy (const AffineTransform& transform) const noexcept
Rectangle< int > toNearestInt () const noexcept
Rectangle< int > toNearestIntEdges () const noexcept
Rectangle< float > toFloat () const noexcept
Rectangle< double > toDouble () const noexcept
Rectangle< TargetType > toType () const noexcept
String toString ()
/* Bool */
bool isEmpty () const noexcept
bool isFinite () const noexcept
bool contains (ValueType xCoord, ValueType yCoord) const noexcept
bool contains (Point< ValueType > point) const noexcept
bool contains (Rectangle other) const noexcept
bool intersects (Rectangle other) const noexcept
bool intersects (const Line< ValueType >& line) const noexcept
bool intersectRectangle (ValueType& otherX, ValueType& otherY, ValueType& otherW, ValueType& otherH) const noexcept
bool intersectRectangle (Rectangle< ValueType >& rectangleToClip)
/* Static */
static Rectangle leftTopRightBottom (ValueType left, ValueType top, ValueType right, ValueType bottom) noexcept
static Rectangle findAreaContainingPoints (const Point< ValueType >* points, int numPoints) noexcept
static Rectangle fromString (StringRef stringVersion)
static bool intersectRectangles (ValueType& x1, ValueType& y1, ValueType& w1, ValueType& h1, ValueType x2, ValueType y2, ValueType w2, ValueType h2) noexcept
< Rectangle 클래스의 크기 >
< Rectangle 객체를 인자로 받는 녀석들 : 다른 클래스의 멤버 함수들 >