2.1 Programs
프로그램이란, 컴퓨터에게 무엇을 할지 (what to do) 설명의 모음이다.
프로그래밍이란, 프로그램을 만드는 행위.
사람이 사람에게 무언가를 명령할때, 사람은 인간의 상식을 통해 얼추 알아듣지만
컴퓨터는 멍청하기때문에, 모든것을 알려주여야한다.
컴퓨터에게 이런 상황을 아주 자세하게 (precisely) 알려주기위해,
특정한 문법을 바탕으로 나온 언어를 프로그래밍 언어 (programming language) 라고 한다.
2.2 The classic first program
아래의 대표적으로 screen 에 "Hello World!" 를 출력하는 코드이다.
#include <iostream>
int main() {
std::cout << "Hello World!";
return 0;
}
모든 C++ 프로그램은, main 함수를 가져야한다.
함수 (function) 는 아래의 4가지 파트로 구성된다.
- Return type : 함수의 반환 type. 위의 코드에서는 int.
- Name : Return type 우측에 나오는 이름, 위의 코드에서는 main.
- Parameter list : () 안에 들어가는것.
- Body : { } 안에 들어가는것
2.3 Compilation
Compile : 사람이 이해할수있는 코드를 기계어로 바꾸는 과정.
Compiler : 컴파일을 해주는 놈
Compiler 는 .cpp 파일들을 .obj 파일로 변환한다.
2.4 Linking
.obj 파일들은 Linker 를 통해 Linking 되어 .exe 파일이 된다.
Compile 과 Linking 과정을 그림으로 표현하면 아래와 같다.
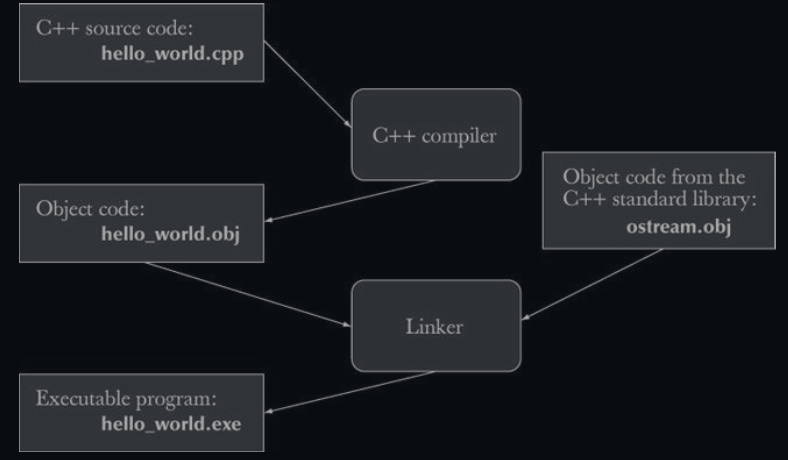
Compile 과 Linking 과정을 거치다보면 error 를 만날수있는데, 종류는 아래와 같다.
- Compile-time error : 컴파일과정에서 발견되는 에러
- Link-time error : Linking 과정에서 발견되는 에러
- Run-time error : 프로그램이 실행중 발견되는 에러
- Logic error
2.5 Programming enviroments
IDE 를 사용하라.
Review
- What is the purpose of the "Hello, World!" program?
- Name the four parts of a function.
- Name a function that must appear in every C++ program.
- In the "Hello, World!" program, what is the purpose of the line return 0; ?
- What is the purpose of the compiler?
- What is the purpose of the #include directive?
- What does a .h suffix at the end of a file name signify in C++?
- What does the linker do for your program?
- What is the difference between a source file and an object file?
- What is an IDE and what does it do for you?
- If you understand everything in the textbook, what is it necessary to practice?
Terms
- //
- <<
- C++
- comment
- compiler
- compile-time error
- cout
- excutable
- function
- header
- IDE
- #include
- library
- linker
- main()
- object code
- output
- program
- source code
- statement
Postscript
programming 을 공부할때, 복잡한것을 만나면 매우 작은 단위로 쪼개어 공부하라. (serious of small steps)
이 공부법은 프로그래밍에만 국한된 이야기가 아니다.